# Modal 模态框 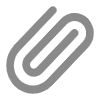
弹出模态框,常用于消息提示、消息确认、在当前页面内完成特定的交互操作。类似于uniapp
官方API uni.showModal(OBJECT)
。
# 平台兼容性
App(vue) | App(nvue) | H5 | 小程序 | VUE2 | VUE3 |
---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ |
# 基本使用
默认情况下,模态框只有一个确认
按钮:
- 请至少要配置弹框的内容参数
content
。 - 通过
ref
调用open()
控制组件的打开。
<template>
<view>
<uv-modal ref="modal" title="标题" content='不知天上宫阙,今夕是何年' @confirm="confirm"></uv-modal>
<button @click="openModal">打开</button>
</view>
</template>
<script>
export default {
methods: {
openModal() {
this.$refs.modal.open();
},
confirm() {
console.log('点击确认按钮');
}
}
}
</script>
# 传入富文本内容
有时候我们需要给模态框的内容,不一定是纯文本的字符串,可能会需要换行,嵌入其他元素等。这时候我们可以使用slot
功能,再结合uni
官方提供的rictText
组件,
就能传入富文本内容了,如下:
<template>
<view>
<uv-modal ref="modal" title="标题" @confirm="confirm">
<view class="slot-content">
<rich-text :nodes="content"></rich-text>
</view>
</uv-modal>
<button @click="openModal">打开</button>
</view>
</template>
<script>
export default {
data() {
return {
content: `<p style="max-height:30px;overflow:auto;">空山新雨后<br>天气晚来秋空山</p>`
}
},
methods: {
openModal() {
this.$refs.modal.open();
},
confirm() {
console.log('点击确认按钮');
}
}
}
</script>
温馨提示
如果想要在模态框中设置滚动内容,可以配合上述富文本使用,设置最大高度和允许滚动属性即可
# 自定义确定按钮
- 一般用于在微信小程序弹窗通过按钮授权等场景。
- 使用
ref
调用close
方法手动关闭弹窗。
<template>
<view>
<uv-modal ref="modal" title="水调歌头" content='不知天上宫阙,今夕是何年' @confirm="confirm">
<template v-slot:confirmButton>
<button type="default" @click="closeModal">自定义按钮</button>
</template>
</uv-modal>
<button @click="openModal">打开</button>
</view>
</template>
<script>
export default {
methods: {
openModal() {
this.$refs.modal.open();
},
closeModal() {
this.$refs.modal.close();
},
confirm() {
console.log('点击确认按钮');
}
}
}
</script>
# 异步关闭
异步关闭只对“确定”按钮起作用,需要设置asyncClose
为true
,当点击确定按钮的时候,按钮的文字变成一个loading动画,此动画的颜色为
confirm-color
参数的颜色,同时Modal不会自动关闭,可以使用ref
调用close
方法手动关闭弹窗,也可以使用ref
调用closeLoading
方法关闭loading动画。
<template>
<view>
<uv-modal ref="modal" title="水调歌头" content='不知天上宫阙,今夕是何年' :asyncClose="true" @confirm="confirm"></uv-modal>
<button @click="openModal">打开</button>
</view>
</template>
<script>
export default {
methods: {
openModal() {
this.$refs.modal.open();
},
confirm() {
console.log('点击确认按钮');
setTimeout(() => {
this.$refs.modal.close();
}, 2000)
}
}
}
</script>
# 控制模态框宽度
可以通过width
属性设置模态框的宽度,不支持百分比,单位px(rpx)
<uv-modal ref="modal" content="不知天上宫阙,今夕是何年" width="500rpx"></uv-modal>
# 缩放效果
默认开启缩放效果,在打开和收起模态框的时候,会带有缩放效果,具体效果请见示例,通过zoom
属性配置
<uv-modal ref="modal" content="不知天上宫阙,今夕是何年" :zoom="false"></uv-modal>
# 完整示例
# API
# Modal Props
参数 | 说明 | 类型 | 默认值 | 可选值 |
---|---|---|---|---|
title | 标题内容 | String | - | - |
content | 模态框内容,如传入slot 内容,则此参数无效 | String | - | - |
confirmText | 确认按钮的文字 | String | 确认 | - |
cancelText | 取消按钮的文字 | String | 取消 | - |
showConfirmButton | 是否显示确认按钮 | Boolean | true | true | false |
showCancelButton | 是否显示取消按钮 | Boolean | false | true | false |
confirmColor | 确认按钮的颜色 | String | #2979ff | - |
cancelColor | 取消按钮的颜色 | String | #606266 | - |
buttonReverse | 对调确认和取消的位置 | Boolean | false | true | false |
zoom | 是否开启缩放模式 | Boolean | true | true | false |
asyncClose | 是否异步关闭,只对确定按钮有效,见异步关闭 | Boolean | false | true | false |
closeOnClickOverlay | 是否允许点击遮罩关闭该组件 | Boolean | true | true | false |
negativeTop | 往上偏移的值,给一个负的margin-top,往上偏移,避免和键盘重合的情况,单位任意,数值则默认为rpx单位 | String | Number | 0 | - |
width | modal宽度,不支持百分比,可以数值,单位px(rpx) | String | Number | 650rpx | - |
zIndex | 弹出层的层级 | Number | String | 10075 | - |
align 1.0.7 | 文本对齐方式 | String | left | left | center | right |
textStyle 1.0.7 | 文本扩展样式 | Object | - | - |
# Modal Methods
事件名 | 说明 |
---|---|
open | 弹出模态框组件 |
close | 关闭模态框组件 |
closeLoading | :asyncClose="true" 异步关闭场景,验证通过则关闭,验证不通过不关闭且按钮恢复加载前的状态等场景,可以调用此方法关闭加载状态 |
# Modal Events
事件名 | 说明 | 回调参数 |
---|---|---|
@confirm | 点击确认按钮时触发 | - |
@cancel | 点击取消按钮时触发 | - |
@close | 关闭模态框组件时触发 | - |
# Modal Slots
名称 | 说明 |
---|---|
default | 传入自定义内容,一般为富文本,见上方说明 |
confirmButton | 传入自定义按钮,用于在微信小程序弹窗通过按钮授权的场景 |