# Subsection 分段器 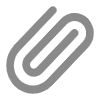
该分段器一般用于用户从几个选项中选择某一个的场景
# 平台兼容性
App(vue) | App(nvue) | H5 | 小程序 | VUE2 | VUE3 |
---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ |
注意
如果在弹窗等场景使用显示异常,需要使用v-if进行控制,详情请参考为什么部分组件在弹出层中显示异常或功能异常 (opens new window)
# 基本使用
- 通过
list
数组参数传递分段的选项,数组元素可为字符串,或者通过keyName
参数传入对象(默认为name
) - 通过
current
属性指定初始化时激活的选项
<template>
<uv-subsection :list="list" :current="current" @change="change"></uv-subsection>
</template>
<script>
export default {
data() {
return {
list: ['未付款', '待评价', '已付款'],
// 或者如下,也可以配置keyName参数修改对象键名
// list: [{name: '未付款'}, {name: '待评价'}, {name: '已付款'}],
current: 1
}
},
methods: {
change(index) {
this.current = index;
}
}
}
</script>
# 模式选择
通过mode
属性设置分段器的模式
- 值为
button
时为按钮类型,默认该值 - 值为
subsection
时为分段器形式
<template>
<uv-subsection :list="list" mode="subsection" :current="current" @change="change"></uv-subsection>
</template>
<script>
export default {
data() {
return {
list: ['未付款', '待评价', '已付款'],
current: 1
}
},
methods: {
change(index) {
this.current = index;
}
}
}
</script>
# 颜色配置
- 通过
activeColor
配置激活选项的文字颜色 - 通过
inactiveColor
配置未激活选项的文字颜色 - 通过
bgColor
配置背景颜色,mode="button"
时有效(默认#eeeeef
)
<template>
<uv-subsection
:list="list"
mode="button"
:current="current"
activeColor="#f56c6c"
inactiveColor="#a9e08f"
bgColor="#f56c6c"
@change="change">
</uv-subsection>
</template>
<script>
export default {
data() {
return {
list: ['未付款', '待评价', '已付款'],
current: 1
}
},
methods: {
change(index) {
this.current = index;
}
}
}
</script>
# 自定义样式
通过custom-style
和custom-item-style
属性可以自定义一些样式,比如设置圆角等,示例如下:
<template>
<uv-subsection
:list="list"
:current="current"
custom-style="height: 60rpx;border-radius: 30rpx;"
custom-item-style="border-radius: 30rpx;"
@change="change"></uv-subsection>
</template>
<script>
export default {
data() {
return {
list: ['未付款', '待评价', '已付款'],
current: 1
}
},
methods: {
change(index) {
this.current = index;
}
}
}
</script>
# 注意事项
如果想通过一个变量绑定current
值,需要在change
事件回调中修改此值,否则可能会由于props
的限制,前后两次设置current
为相同的值,
而导致无法通过修改current
值触发分段器的变化。
<template>
<uv-subsection :list="list" :current="current" @change="change"></uv-subsection>
</template>
<script>
export default {
data() {
return {
list: ['未付款', '待评价', '已付款'],
current: 0
}
},
methods: {
change(index) {
this.current = index;
}
}
}
</script>
# 完整示例
# API
# Subsection Props
参数 | 说明 | 类型 | 默认值 | 可选值 |
---|---|---|---|---|
list | 选项的数组,形式见上方"基本使用" | Array | - | - |
keyName | 从list 元素对象中读取的键名 | String | name | - |
current | 初始化时默认选中的选项索引值 | String | Number | 0 | - |
activeColor | 激活时的颜色 | String | #3c9cff | - |
inactiveColor | 未激活时的颜色 | String | #303133 | - |
mode | 模式选择,见上方"模式选择"说明 | String | button | button | subsection |
fontSize | 字体大小,单位px(rpx) | String | Number | 12 | - |
bold | 激活选项的字体是否加粗 | Boolean | true | true | false |
bgColor | 组件背景颜色,mode 为button 时有效 | String | #eeeeef | - |
customStyle | 自定义外部样式 | String | Object | - | - |
customItemStyle | 自定义bar样式 | String | Object | - | - |
# Subsection Events
事件名 | 说明 | 回调参数 |
---|---|---|
change | 分段器选项发生改变时触发 | index:选项的index索引值,从0开始 |