# Picker 选择器 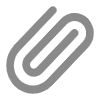
此选择器用于单列,多列,多列联动的选择场景。
# 平台兼容性
App(vue) | App(nvue) | H5 | 小程序 | VUE2 | VUE3 |
---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ |
# 基本使用
- 通过
ref
调用open()
控制组件的打开。 - 都通过传入数组
columns
配置选择项。
注意
columns参数中是数组中嵌套数组:columns: [ ['中国', '美国', '日本'] ]
,否则显示异常
<template>
<view>
<uv-picker ref="picker" :columns="columns" @confirm="confirm"></uv-picker>
<button @click="openPicker">打开</button>
</view>
</template>
<script>
export default {
data() {
return {
columns: [
['中国', '美国', '日本']
]
}
},
methods: {
openPicker() {
this.$refs.picker.open();
},
confirm(e) {
console.log('confirm', e);
}
}
}
</script>
# 多列模式与多列联动
此模式通过传入columns
参数,此参数为二维数组,通过change
事件完成联动操作。
<template>
<view>
<uv-picker ref="picker" :columns="columns" @confirm="confirm" @change="change"></uv-picker>
<button @click="openPicker">打开</button>
</view>
</template>
<script>
export default {
data() {
return {
columns: [
['中国', '美国'],
['深圳', '厦门', '上海', '拉萨']
],
columnData: [
['深圳', '厦门', '上海', '拉萨'],
['得州', '华盛顿', '纽约', '阿拉斯加']
]
}
},
methods: {
openPicker() {
this.$refs.picker.open();
},
confirm(e) {
console.log('confirm', e);
},
change(e) {
const { columnIndex , index} = e
if (columnIndex === 0) {
this.$refs.picker.setColumnValues(1, this.columnData[index])
}
}
}
}
</script>
# 省市区三级联动
需要用到该示例中的regions.json文件,可以到uv-ui (opens new window)项目中相应目录找到
注意:uvui项目中提供的regions.json只做演示数据,不一定准确,开发者可以使用其他更准确的数据,只要格式一样即可
<template>
<view>
<uv-picker ref="picker" :columns="addressList" :loading="loading" keyName="name" @change="change" @confirm="confirm">
</uv-picker>
<button @click="open">打开</button>
</view>
</template>
<script>
export default {
data() {
return {
loading: true,
provinces: [], //省
citys: [], //市
areas: [], //区
pickerValue: [0, 0, 0],
defaultValue: [3442, 1, 2]
}
},
created() {
this.getData();
},
computed: {
addressList() {
return [this.provinces, this.citys, this.areas];
}
},
methods: {
getData() {
uni.request({
method: 'GET',
url: '/static/uvui/example/regions.json',
success: res => {
const { statusCode, data } = res
if (statusCode === 200) {
console.log('获取的数据:', res);
this.provinces = data.sort((left, right) => (Number(left.code) > Number(right.code) ? 1 : -1));
console.log(this.provinces)
this.handlePickValueDefault()
setTimeout(() => {
this.loading = false
}, 200)
}
}
})
},
handlePickValueDefault() {
// 设置省
this.pickerValue[0] = this.provinces.findIndex(item => Number(item.id) === this.defaultValue[0]);
// 设置市
this.citys = this.provinces[this.pickerValue[0]]?.children || [];
this.pickerValue[1] = this.citys.findIndex(item => Number(item.id) === this.defaultValue[1]);
// 设置区
this.areas = this.citys[this.pickerValue[1]]?.children || [];
this.pickerValue[2] = this.areas.findIndex(item => Number(item.id) === this.defaultValue[2]);
// 重置下位置
this.$refs.picker.setIndexs([this.pickerValue[0], this.pickerValue[1], this.pickerValue[2]],true);
},
change(e) {
if (this.loading) return;
const { columnIndex, index, indexs } = e
// 改变了省
if (columnIndex === 0) {
this.citys = this.provinces[index]?.children || []
this.areas = this.citys[0]?.children || []
this.$refs.picker.setIndexs([index, 0, 0],true)
} else if (columnIndex === 1) {
this.areas = this.citys[index]?.children || []
this.$refs.picker.setIndexs(indexs,true)
}
},
open() {
this.$refs.picker.open();
this.handlePickValueDefault()
},
confirm(e) {
console.log('确认选择的地区:', e);
uni.showToast({
icon: 'none',
title: `${e.value[0].name}/${e.value[1].name}/${e.value[2].name}`
})
}
}
}
</script>
# 加载状态
由于需要多列联动,此模式和上面的"多列模式"基本相同,在加载之前将loading
设置为true
,数据获取完成之后再置为false
即可。
<template>
<view>
<uv-picker ref="picker" :loading="loading" :columns="columns" @change="change"></uv-picker>
<button @click="openPicker">打开</button>
</view>
</template>
<script>
export default {
data() {
return {
loading: false,
columns: [
['中国', '美国'],
['深圳', '厦门', '上海', '拉萨']
],
columnData: [
['深圳', '厦门', '上海', '拉萨'],
['得州', '华盛顿', '纽约', '阿拉斯加']
]
}
},
methods: {
openPicker() {
this.$refs.picker.open();
},
confirm(e) {
console.log('confirm', e);
},
change(e) {
console.log('change');
const { columnIndex, index } = e;
if (columnIndex === 0) {
this.loading = true;
setTimeout(()=>{
this.$refs.picker.setColumnValues(1, this.columnData[index]);
this.loading = false;
},2000)
}
}
}
}
</script>
# 自定义选项值
参数columns
可以传入自定义选项值,可以通过keyName
参数控制对应的显示属性。
<template>
<view>
<uv-picker ref="picker" :columns="columns" keyName="label" @confirm="confirm"></uv-picker>
<button @click="openPicker">打开</button>
</view>
</template>
<script>
export default {
data() {
return {
columns: [
[{
label: '雪月夜',
// 其他属性值
id: 2021
// ...
}, {
label: '冷夜雨',
id: 804
}]
]
}
},
methods: {
openPicker() {
this.$refs.picker.open();
},
confirm(e) {
console.log('confirm', e);
}
}
}
</script>
# 自定义颜色
通过color
和activeColor
属性分别设置文本颜色和文本选中颜色
<template>
<view>
<uv-picker ref="picker" :columns="columns" color="red" activeColor="blue" @confirm="confirm"></uv-picker>
<button @click="openPicker">打开</button>
</view>
</template>
<script>
export default {
data() {
return {
columns: [
['中国', '美国', '日本']
]
}
},
methods: {
openPicker() {
this.$refs.picker.open();
},
confirm(e) {
console.log('confirm', e);
}
}
}
</script>
# 默认值
- 通过defaultIndex设置:
此组件的所有模式,都可以设置默认值,通过defaultIndex
数组参数配置,数组元素的0表示选中每列的哪个值(从0开始),下面分别对几种模式进行说明:
注意: defaultIndex
数组的长度,必须与列数相同,否则无效。
- 单列模式
如设置defaultIndex
为[1]
表示默认选中第2个(从0开始),[5]
表示选中第6个。
- 多列模式
如设置defaultIndex
为[1, 3]
表示第一列默认选中第2个,第二列默认选中第4个。
- 通过setIndexs()方法设置:
也可以使用this.$refs.picker.setIndexs([1],true)
进行设置,第一个参数与defaultIndex
规则一样。
# 完整示例
# API
# Picker Props
参数 | 说明 | 类型 | 默认值 | 可选值 |
---|---|---|---|---|
columns | 设置每一列的数据,见上方说明 | Array | - | - |
keyName | 自定义columns 参数中需要展示的text 属性键名 | String | - | - |
showToolbar | 是否显示顶部的操作栏 | Boolean | true | true | false |
title | 顶部中间的标题 | String | - | - |
round 1.0.10 | 弹窗圆角 | String | Number | 0 | - |
loading | 加载状态 | Boolean | false | true | false |
itemHeight | 各列中,单个选项的高度 | String | Number | 44 | - |
cancelText | 取消按钮的文字 | String | 取消 | - |
confirmText | 确认按钮的文字 | String | 确认 | - |
cancelColor | 取消按钮的颜色 | String | #909193 | - |
confirmColor | 确认按钮的颜色 | String | #3c9cff | - |
color 1.0.5 | 文本颜色 | String | #606266 | - |
activeColor 1.0.5 | 选中文本颜色 | String | #606266 | - |
visibleItemCount | 每列中可见选项的数量 | String | Number | 5 | - |
closeOnClickOverlay | 是否允许点击遮罩关闭选择器 | Boolean | true | true | false |
closeOnClickConfirm | 是否允许点击确认关闭选择器 | Boolean | true | true | false |
defaultIndex | 各列的默认索引。如果设置该值不生效,请使用下方的setIndexs() 方法 | Array | - | - |
immediateChange | 是否在手指松开时立即触发change 事件。若不开启则会在滚动动画结束后触发change 事件,只在微信2.21.1 及以上有效 | Boolean | true | true | false |
# Picker Methods
名称 | 说明 |
---|---|
open | 弹出颜色选择组件 |
close | 关闭颜色选择组件 |
setIndexs | 设置对应列的选择值,eg:this.$refs.picker.setIndexs([1],true) |
setColumnValues | 多列联动时需要用到,见上方用法 |
# Picker Events
事件名 | 说明 | 回调参数 |
---|---|---|
@confirm | 点击确定按钮触发 | Array: 见上方"回调参数"部分说明 |
@change | 当选择值变化时触发 | Array: 见上方"回调参数"部分说明 |
@cancel | 点击取消按钮触发 | - |
@close | 关闭选择器时触发 | - |